How to Read a Text File with Go
Last Update: Jun 7, 2024
I wrote a book! Check out A Quick Guide to Coding with AI.
Become a super programmer!
Learn how to use Generative AI coding tools as a force multiplier for your career.
If you want to read text data in Go, it’s incredibly easy. In this guide I’ll show you my favorite way to open and read text files with Go.
Note: If you’d rather watch a video for this tutorial, it’s available here: How to Read a Text File with Go
The Text File We’re Using
I’ll be using a (fake) customer list that’s in a CSV format. It looks like this:
I used my favorite fake data generator Mockaroo for this file. I highly suggest checking out their site.
You can download the exact file I’m using here.
We have
- id
- first_name
- last_name
- gender
- ip_address
As fields in this file. We want to read this file line by line, and break up the line (string) into separate fields and display them. Let’s get started.
Creating the Go Program
Let’s create a text file named textviewer.go
and open it up.
Let’s declare our package on the top line:
package main
and create our main function:
func main() {
}
This will be our main file for the Go program we’re about to write.
Open The Text File
Before we can parse data, we need to open the text file. Below the first line (package main) add the following imports:
import (
"os"
"bufio"
"fmt"
"strings"
)
This will allow us to use pre-written code to open the text file and other functions in this program.
Inside our main function, add this line:
file, ferr := os.Open("customerlist.csv")
This will open up the customerlist.csv
file using os.Open(). We will use the file
handler to work with the file. If the file cannot be opened, the error will be stored in the ferr
variable so we can display it.
Next, we will check for that error and stop the program if it exists. If we can’t open the file, this program will not do what we want it to, so we should close it.
if ferr != nil {
panic(ferr)
}
Read the Data
Now we need to read the data from the text file. We will use a Bufio scanner. This is a buffered scanner from the Go Standard Library that reads the data from a file and loads it into a buffer. It does a lot with a small amount of code.
scanner := bufio.NewScanner(file)
We’ll use scanner
or read the data in file
, which we’ve already loaded.
Now, let’s parse the data.
Parse the Data
We have the spanner started up, and now we need to access it. We will use a for loop to do that.
for scanner.Scan() {
}
The scanner will read the text file line by line. These statements in this loop will run until there are no more lines in the text file. Then it will stop.
Let’s read the line into a string.
line := scanner.Text()
Then, we’ll split the line into an array. We’ll split it by commas, so each comma means we create a new field in the array.
We’ll use the strings library to split the string at each comma.
items := strings.Split(line, ",")
This will create an array of items we will display on our screen in the next step.
Display the Data
Next, we will display the data on our screen.
Since items
is a string array, we can access the data directly with an indexer.
fmt.Printf("Name: %s %s Email: %s\n", items[1],items[2],items[3])
This displays each row’s first name, last name, and email address.
Let’s add a separator to go after each item.
fmt.Println("------")
}
Now we’re ready to run the Program and test it out.
Run the Program
There are two ways to run the program. You can type in:
go run textviewer.go
or you can build it and run it:
go build textviewer.go
./textviewer
Note: In Windows it will be textviewer.exe
You should see something that looks like this:
And you did it! You can see the output as expected.
You can format this data however you want. Change the print statement to this:
fmt.Printf("Name: %s %s \nEmail: %s\nIP Address: %s\n", items[1], items[2], items[3], items[5])
Here we’ve added some line breaks and an IP address to the display.
The possibilities are endless!!
Summary
In this tutorial, we learned how to:
- Open a file
- Read CSV data
- Format this data
- Display it at the prompt
I hope this has helped you in your journey to learn Go. Bookmark this blog for more Go Tutorials.
Questions, comments? Let me know!
Here’s the Video Version of this Tutorial:
Subscribe to my Channel!
Want to become an expert Go programmer?
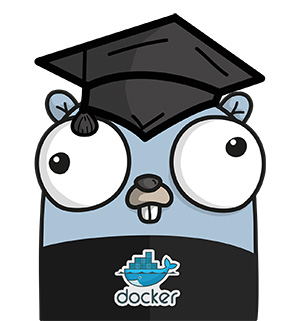
You can boost your skills in a few weekends by taking these high quality Go courses taught by top experts!
We have over 30 hours of Go courses you can take now! You can try it for 10 days free of charge.