Properties vs Fields in C#
Last Update: Jun 7, 2024
Today’s sponsor is Depot. Depot is a remote container build service that’s half the cost of GitHub Actions runners and up to 40x faster than other build services. Try it for free.
Note: Updated for 2023
One of the areas that cause a lot of confusion for new C# developers is the idea of properties and fields. It’s an easy thing to mess up or get confused about. Here are some general guidelines to help you decide how to use these members in your project.
Fields: The Basics
Fields are variables that belong to a Class or a Struct. They’re like little storage containers that hold the data for your class.
Let’s consider a typical Jeremy example: a class called Car with fields for make and model.
Here’s what it might look like:
class Car
{
public string make;
public string model;
}
Now, you can create a new Car object and set the make and model fields like this:
Car car1 = new Car();
car1.make = "Ford";
car1.model = "Mustang";
Seems straightforward, right? Well, not so fast! Fields have a small problem: they expose the class’s internal implementation details to the outside world. This can lead to all sorts of issues, like data corruption or misuse of class members.
Properties: A Better Alternative
Properties allow you to encapsulate (or wrap) your fields with special methods called accessors. These accessors control how the data is read and written, giving you better control over your class’s internals.
Properties come in two flavors: getters and setters. A getter is a method that returns the value of a field, while a setter is a method that assigns a new value to the field. Here’s our previous Car class, this time with properties:
class Car
{
private string make;
private string model;
public string Make
{
get { return make; }
set { make = value; }
}
public string Model
{
get { return model; }
set { model = value; }
}
}
Now, instead of directly accessing the fields, we use the properties:
Car car1 = new Car();
car1.Make = "Toyota";
car1.Model = "Camry";
You might be thinking, “Hey, this looks almost the same as before!” And you’re right. The key difference is that we now have control over how the data is accessed and modified. Plus, we can add extra logic to the getters and setters, like validation or transformation.
When should I use a property?
In general, you should use properties if you need them to look and behave like a variable. Properties give you a level of abstraction to change the fields while not affecting how a class uses them.
Here are some basic rules:
- Should be quick to read - reading a property should be just as fast as reading a variable. If heavy calculations are involved or you need to go to a database every time it’s needed, this isn’t the best way to use a property.
- Client code should be able to read its value. - Write only properties are best reserved for methods.
- Reading and writing to a property should not have any side effects - You should have no unexpected actions or side effects when working with this value. Changing this value should produce the results expected (like a color on a web page, for instance), but changes should not be able to break the functionality of your program.
- Properties should require an order - You should be able to set the properties in any order. You should not have any errors in a property if another property has not been set. This creates a dependency chain that can make your program unpredictable.
- Validation and other logic is fine - you can encapsulate logic in properties, and adding an additional level of validation here is fine, if not recommended in some cases.
- Read it a million times with the same result - You should be able to read the property multiple times with the same result. If you have a value that’s constantly changing, like how much gas is in the gas tank, a
GetGasLevel()
method would be better. Use a property if you want to store values that don’t often change, like the number of gallons the tank holds.
Auto-Implemented Properties: The Shortcut
C# developers are lazy (I include myself in that) and love finding ways to make things easier. That’s why C# introduced auto-implemented properties, which allow you to create a property without writing the getter and setter code. The compiler takes care of the details for you. Here’s our Car class, now with auto-implemented properties:
class Car
{
public string Make { get; set; }
public string Model { get; set; }
}
That’s it! The code is cleaner, and we still have all the benefits of properties. Note that you can still add custom logic to your getters and setters if needed, but for simple cases, auto-implemented properties are a fantastic time-saver.
Summary
Fields are basic variables that hold data in a class, while properties provide a safer and more flexible way to access and modify that data. By using properties, you can keep your code clean and maintain better control over how data is managed in your class.
I hope that clarifies the name vs. property question when dealing with objects. Remember, there are no hard rules here, so if you really need to violate these guidelines you can. Remember some changes may make extension or interfacing difficult.
If you want to ramp up your C# and become a .NET wizard, check out the C# course path on Pluralsight you can go from beginner to expert in no time!
Questions, comments? Let me know!
Can you beat my SkillIQ Score in C#??
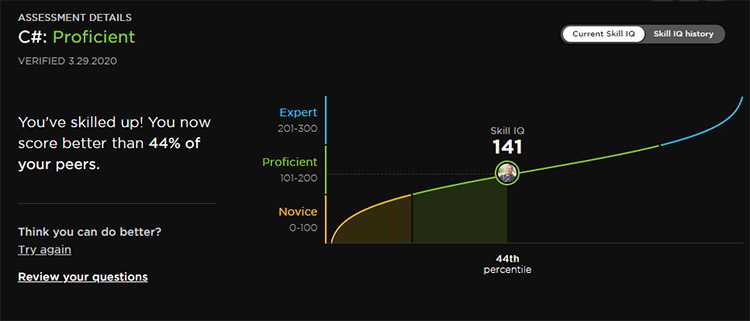
I think you can! Take the C# test here to see where you stand!
Today’s sponsor is Depot. Depot is a remote container build service that’s half the cost of GitHub Actions runners and up to 40x faster than other build services. Try it for free.