How Do I Install OpenCV for Python in Arch Linux?
Last Update: Mar 8, 2023

I’ve been playing around with OpenCV and Python lately. It’s a ton of fun. I often bounce around from machine to machine. I struggled a bit getting my OpenCV stuff to work with Arch. Then I went to another machine and decided to find the easiest way to set it up, and that’s what this tutorial is about.
This tutorial doesn’t cover building OpenCV from source but shows the quickest way to get up and running with OpenCV and Python in Arch.
Get Python Set Up
Ok, this one should be obvious, but you’ll need Python 3 installed.
If for some reason it isn’t, it’s easy:
sudo pacman -S python
Next, you’ll need to install VirtualEnv
sudo pacman -S python-virtualenv
This allows you to create virtual environments, which I highly recommend.
Create a Virtual Environment
It’s incredibly easy to set up a Python Virtual Environment in Arch. I’ll set up a folder named “cvtest”.
virtualenv cvtest
cd cvtest
source bin/activate
Now you have your environment set up, and you can use pip to install what you need.
Install Some Libraries
pip install numpy
The first time running pip, you may get a message like this:
Follow the instructions to upgrade pip, and it should work fine.
Install the OpenCV for Python library:
pip install opencv-python
Now you should be ready to go!
Test It Out
Here’s a quick Python app I put together to test out OpenCV. It creates a canvas and builds an old school gradient. Then it grabs your CPU and Memory Info. Then it draws a rectangle, drops the text in, and writes it all to an image. You can get the source from GitHub if you want.
import numpy as np
import cv2
import os
import random
canvas = np.zeros((255, 510, 3), dtype="uint8")
for x in range(255):
cv2.line(canvas, (x, 0), (x, 255), (0, 0, x))
cv2.line(canvas, (509-x, 0), (509-x, 255), (0, 0, x))
f = os.popen('cat /proc/cpuinfo | grep \'model name\' | uniq | cut -c 14-')
m = os.popen('cat /proc/meminfo | grep MemFree')
cpu = f.read()[:-1]
memfree = m.read()[:-1]
print(cpu)
print(memfree)
cv2.rectangle(canvas,(45,156),(450,95),(255,255,255),-1)
font = cv2.FONT_HERSHEY_PLAIN
cv2.putText(canvas,cpu,(60,123), font, 1,(0,0,0),2,cv2.LINE_AA)
cv2.putText(canvas,memfree,(60,145), font, 1,(0,0,0),2,cv2.LINE_AA)
cv2.imshow("Canvas", canvas)
cv2.imwrite("newimage.jpg", canvas)
cv2.waitKey(5000)
Now, you should be able to run this file, and see the following:
Congrats! You’ve got it installed!
Summary
I found a lot of conflicting information online for using OpenCV and Python in Arch. I found tutorials that showed a bunch of different ways to compile stuff, use AURs, or use pip after installing a bunch of stuff.
I found this way to be the fastest way to get up and running in Arch Linux. You may run into a case where you need a feature and have to compile OpenCV, but for most basic stuff, you should be able to use this “pip within an environment” method.
As always, Go build some cool stuff, and if you have questions or comments let me know!
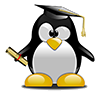
Want to learn more about Linux? Of course you do. Check out this Linux Fundamentals course. You can sign up for a free trial here and take it today!