How to write text files in C#
Last Update: Jun 7, 2024
Today’s sponsor is Depot. Depot is a remote container build service that’s half the cost of GitHub Actions runners and up to 40x faster than other build services. Try it for free.
So you want to know how to write a text file in C#? It’s actually super easy. This tutorial explains how.
Note: I wrote this article over 10 years ago, and I’m re-writing it. Let me know what you think it should include.
using System;
using System.IO;
public class FileWriter
{
public static void Main()
{
StreamWriter OurStream;
OurStream = File.CreateText("c:\examples\writer\test.txt");
OurStream.WriteLine("This is a line of text");
OurStream.Close();
Console.WriteLine("Created File!");
}
}
When you run this code from your IDE (F5 Button) it will write a text file in the C:\examples\filewriter directory.
How to start
Open up Visual Studio Express, and select File->New Project
Create a new project called “filewriter”, using the following options:
Create a new console application and copy and paste the code from above in. I’ll explain how each step works.
At the top of the file, you have the following two lines:
using System;
using System.IO;
What this does is include the System and System.IO classes, so you can use methods from them. Without these lines, the compiler will not be able to fetch the code for the methods we’ll be using.
public class FileWriter
{
This code creates a new object (or class) that will run when compiled. For more details on classes and objects, check out this page.
For this application, every task is executed within this class, within the brackets.
public static void Main()
{
These lines create yet another loop, that tell the compiler to run everything within the bracket automatically on load, using the Main() method. Anything loaded in this method will be run when the main executable is run.
Next, we want to create a StreamWriter object. This class creates a textwriter to write characters to a stream. This is commonly used for text file operations. (more info on StreamWriter)
StreamWriter OurStream;
Then, we actually create the file, using the CreateText Method from the File class:
OurStream = File.CreateText("c:\examples\filewriter\test.txt");
Then, we write a string of text to the stream:
OurStream.WriteLine("This is a line of text");
You can really work with this a lot, there are tons of ways to expand on this. You can take text from another file using a streamreader, or text from a database, xml file, or whatever you want. The possibilities are endless.
When we’re done writing, we close the file with the following line:
OurStream.Close();
This closes the stream. This is very important, if you leave too many streams open, it will take up valuable resources. This is especially important in web apps (which this app is not, but something you should keep in mind).
The last line we just do just to let you know that we done.
Console.WriteLine("Created File!");
When you compile and run your program, you should see this:
Questions? Comments? Discuss it here
Can you beat my SkillIQ Score in C#??
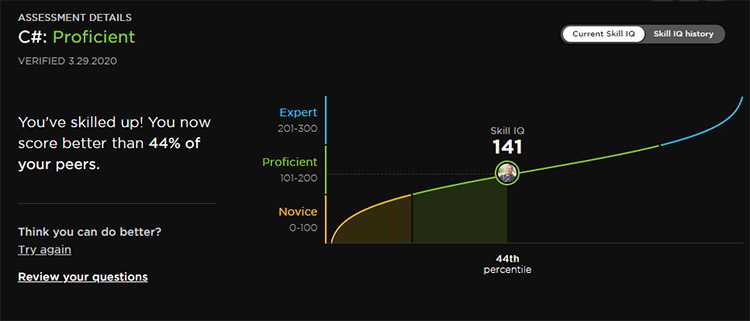
I think you can! Take the C# test here to see where you stand!
Can you beat my SkillIQ Score in C#??
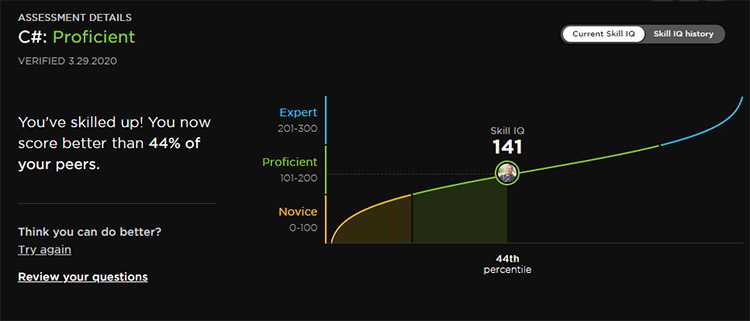
I think you can! Take the C# test here to see where you stand!
Today’s sponsor is Depot. Depot is a remote container build service that’s half the cost of GitHub Actions runners and up to 40x faster than other build services. Try it for free.